Highlighting a GridView row is more than just to make it look good. It helps to identify and focus on a particular row, since the GridView shows many rows of data at a time. In this article we will discuss on how to highlight a GridView row on MouseOver event.
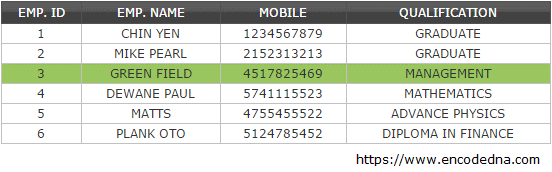
The Markup
<!DOCTYPE html>
<html>
<head>
<title>GridView Row Highlight on MouseOver</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<!-- ADD A GRIDVIEW CONTROL. -->
<asp:GridView ID="GridView"
Font-Names="Arial"
Font-Size="0.75em"
CellPadding="5"
CellSpacing="0"
ForeColor="#333"
AutoGenerateColumns="False"
onrowdatabound="GridView_RowDataBound"
runat="server">
<HeaderStyle BackColor="#989898" ForeColor="white" />
<Columns>
<asp:TemplateField HeaderText ="Emp. ID">
<ItemTemplate>
<asp:Label ID="lblEmpID" runat ="server" Text ='<%# Eval("EmpID") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText ="Emp. Name">
<ItemTemplate>
<asp:Label ID="lblEmpName" runat ="server" Text ='<%# Eval("EmpName") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText ="Mobile">
<ItemTemplate>
<asp:Label ID="lblMobile" runat ="server" Text ='<%# Eval("Mobile") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText ="Qualification">
<ItemTemplate>
<asp:Label ID="lblQua" runat ="server" Text ='<%# Eval("Qualification") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</div>
</form>
</body>
The Script
<script>
// SCRIPT FOR THE MOUSE EVENT.
function MouseEvents(objRef, evt) {
if (evt.type == "mouseover") {
objRef.style.cursor = 'pointer';
objRef.style.backgroundColor = "#EEEED1";
}
else {
if (evt.type == "mouseout") objRef.style.backgroundColor = "#FFF";
}
}
</script>
</html>
The Script
<script>
// SCRIPT FOR THE MOUSE EVENT.
function MouseEvents(objRef, evt) {
if (evt.type == "mouseover") {
objRef.style.cursor = 'pointer';
objRef.style.backgroundColor = "#EEEED1";
}
else {
if (evt.type == "mouseout") objRef.style.backgroundColor = "#FFF";
}
}
</script>
</html>